Overview
Rental Car Manager (RCM) provides a Web API through which you are able to use to build a set of booking steps which you can plug into your website. This API also allows for Agents to have access and search for availability of vehicles and to make booking requests.
The functions available within the Web API are:
- Search for availability of vehicles for a booking request.
- Obtain a listing of all of the relevant mandatory and optional fees for a booking request.
- Create a quote or a booking inside RCM.
- Save either credit card or payment information against the booking.
Who this is for?
This document gives an overview of the steps required to make a booking. It is intended for web developers who will be creating booking pages from scratch, or heavily customising our provided sample booking pages. If you are confident in making AJAX or server-side API calls and manipulating returned datasets, for selection and display, then this is for you.
Who is this not for?
If you are not confident in building a booking module from scratch, or if you are short on time/resources, we
can provide you with a working solution “out of the box”. Please contact RCM Support (support@rentalcarmanager.com) to enquire about our RCM-hosted web booking steps.Developer Sandbox Area
The first thing you need to do is contact RCM Support and ask for a Sandbox Login.
If you are an agency working with one or more companies, we will need the company to set you up as an Agency within their RCM system first.
The Sandbox Area allows you to download sample code, test all the different API methods, and access and test your Live API Key.
API Credentials
Every API request requires two pieces of information that is unique to you, as an API user.
- API Key
The API key identifies you to RCM. It is passed with every request as part of the querystring.
If you are calling the Web API it should be https://apis.rentalcarmanager.com/booking/v3.2/?apikey=<apikey>
If you are calling the Agent API it should be https://apis.rentalcarmanager.com/agent/booking/v3.2/?apikey=<apikey> - Shared Secret
The shared secret is used to encrypt the main data or body of the request, and pass this value along with the request. The encrypted data is known as the “Signature”. Upon receiving an API request, RCM will derive our own signature using your shared secret to ensure that we have a match. See ‘Request Signing’ below for more details.
Please note that Rental Car Manager has many servers. The actual <apiserver> for accessing live data varies depending on the rental car company that you are integrating with. This will be provided to you
from the sandbox area. Tests from within the sandbox area will be against our test server.
If you are an agent that connects to more than one RCM company, you will be given one API Key/Shared Secret pair for each company. Note that the <apiserver> may be different for each company.
Request Structure
URL
Every request to our v3.2 API has the same url:
https://<apiserver>/api/3.2/<apikey>
The values for <apiserver> and <apikey> are available in the Sandbox Area.
- For server-side requests, the Request Header “signature” should contain the value for the HMACSHA256-encrypted request body.
- For client-side requests (javascript/jquery/ajax), do not use the Request Header “signature”.
See more information about this in the “Request Signing” section below.
Request Body
The request body is a JSON structure, which includes the method name. For the simple API requests, the method may be the only parameter required, e.g.
{“method”:”step1”}
For more complex methods, there will be more data, e.g.
{"method":"search","pickuplocationid":4,"dropofflocationid":4,"pickupdate":"10/04/2018","pickuptime":"11:35","dropoffdate":"17/04/2018","dropofftime":"12:35","ageid":9,"vehiclecategorytypeid":1,"campaigncode":""}
- For server-side requests, the entire request body should be this JSON data.
- For client-side requests (javascript/jquery/ajax), this JSON data should be posted as form data with the form-field name “request”. The entire JSON data value should then be encrypted using the Shared Secret, and added to a second form-field named “signature”. See more information about this in the next “Request Signing” section.
Request Signing
All users of the API will be issued with a shared secret, which is an alphanumeric value. This is not the same value as the API key. This shared secret is used to sign all requests using HMACSHA256 encryption,
the resulting value is called the “signature”.
IMPORTANT: This shared secret value MUST be kept on your server and not sent out to the browser in HTML or javascript.
There are two ways that you can use the shared secret to sign the request:
- Client-Side (javascript/jquery/ajax): Add Signature as a Form value in the Request Body: This is the method to use if you are making the request to our API from javascript in the browser, i.e. a client-side request.
OR
- Server-Side: Add Signature to Request Header: This is a more straight-forward way to sign the request, but you can only use this method if you are calling our API directly from your server, not from javascript in the browser.
Client-Side: Adding the Signature as a Form value in the Request Body
This is the best method to use if you are making the request to our API from javascript in the browser, i.e. a client-side request. This is because the alternative method (adding the signature to the request header) may be denied by the browser security rules.
- You will need to provide a server-side method on your own server (the same host where your client pages are hosted) to return the signed hash value and add it to the request form.
Here is a VB.Net version of this method (in a file named “signRequest.ashx”):
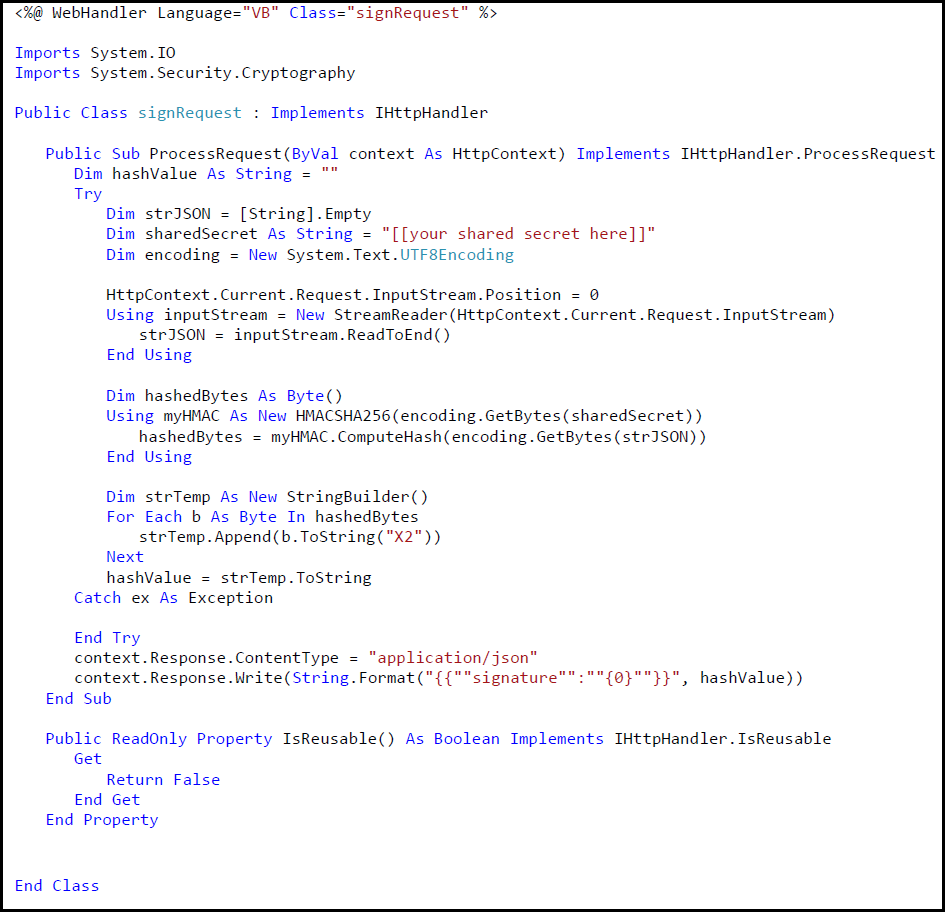
Here is a PHP version of this method (in a file named “signedRequest.php”):

- You can then call this method from your javascript. The data to be signed is the entire JSON request value. For e.g. {“method”:”step1”} or {"method":"vaultentry","reservationref":"123456ABCDEF","data":"vault response data goes here"}
For more info please see each method’s specific data requirements.
Here is a sample javascript ajax call, using the “signRequest.ashx” .Net page as the signing method, and calling our “step1” API method:
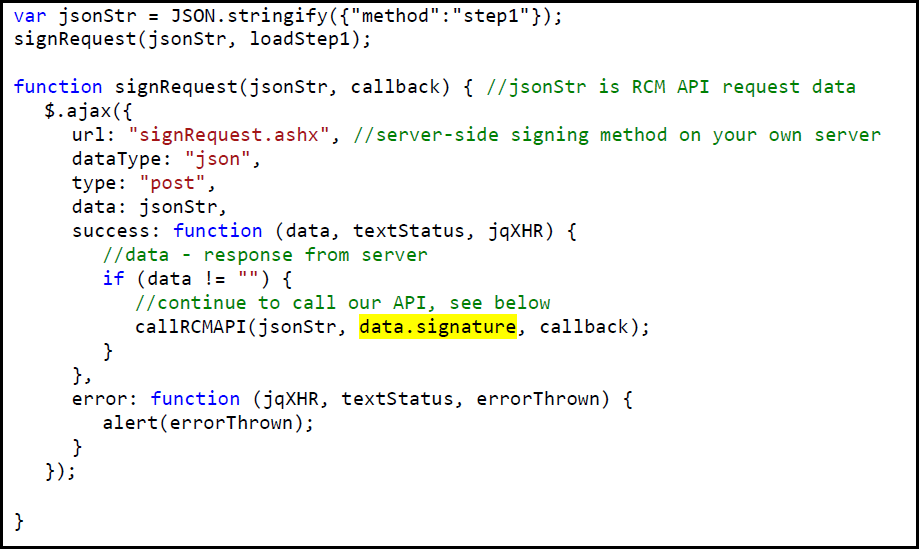
- Use the signed value and add it to the API request as a form value "signature":
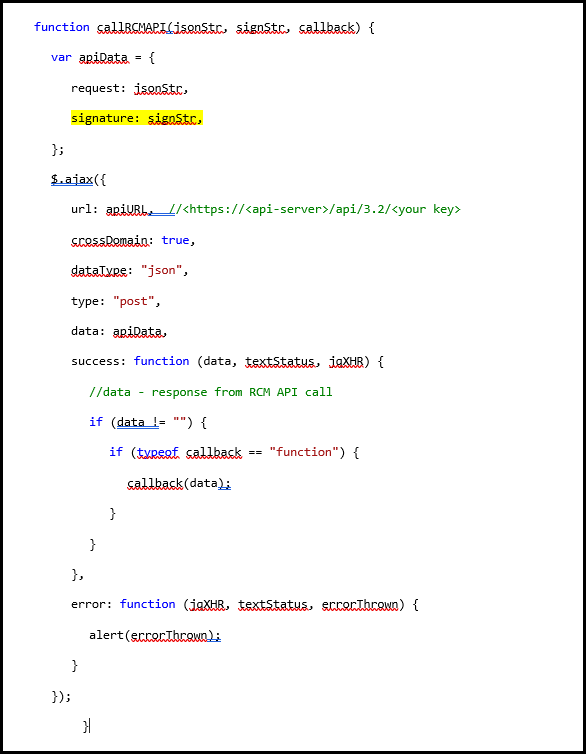
If you are calling our API directly from your web server, i.e. not from a browser, you can choose either to submit the request & signature values as form data (see Client-Side method above), OR you can add the
signature as a Request Header. Adding it as a request header is the recommended method.
To create the signature:
- Get the API request input data. The data to be signed is the entire JSON request value. For e.g. {“method”:”step1”} or {"method":"vaultentry","reservationref":"123456ABCDEF","data":"vault response data goes here"}. For more info please see each method’s specific data requirements.
- Use the hash secret to sign the data using HMACSHA256.
- Add the hex encoded result to the header of the request as in a header value named “signature”.
Sample VB Code
Sample C# Code
Add the hash result to the request header as:
signature: <hash>
PHP Example
To create the hash:
Example:
Curl request:
Response Structure
The response from each API method call is in JSON format, and is in the general structure below:
{"status":"OK", "error": "", "results":{<results here>},"ms":100,"issues":[]}
status: either “OK” or “ERR”. If “ERR”, then refer to the error field below for more information
error: Empty if no errors encountered, otherwise a description of the error. E.g. “Err - Step3: Parameter[carsizeid]. Invalid value [0] expecting valid carsizeid.”
results: Results from the method call are output here. It could be a few values e.g. {"paymentsaved":true,"emailsend":false}, or it could be a large series of datasets e.g. the method “search” returns a large volume of data including datasets named “locations”, “officetimes”, “categorytypes”, “availablecars”, etc. An empty dataset could be a valid result.
ms: The length of time, in milliseconds, that it took to process the request RCM’s server. Examining this value if you are getting slow requests may help pinpoint where the delay is.
issues: Any warnings about the API request that didn’t prevent the request from executing. For example, an invalid value for a non-mandatory field, or where the main request (e.g. booking) succeeded but a secondary function failed (e.g. send email confirmation after booking).
Demo & Sample Code
Demo link
Sample Code & Testing
You can access each of the above options from inside the Sandbox by using the menu on the left-side of the screen, see below.
You will need a username and password to be able to log in to the sandbox area and download the
sample code. Please contact RCM Support (support@rentalcarmanager.com)
if you do not have sandbox credentials.Dataset Reference
All of the datasets are defined in our sandbox area under the API Test Area.
Notes:
- All fieldnames are lower case! Make sure you use lower case to access the correct field.
- Please note that <int_id> is not the same as <int>, <int_id> refers to an integer value that reflects the ID within the RCM system for that particular dataset, and in case this value is 0 that means it is valid for all.
Example:
If we have the next Driver’s age table within the system:
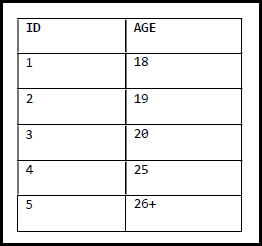
An age_id of 4 equals an age of 25.
Field Types:
Possible field types / options are displayed in the dataset definition as <option1/option2/...>.
<True/False> means we have only 2 possibilities either
- True
- False
<0/1> means we have only 2 possibilities either
- 0
- 1
<Sunday/Monday/Tuesday/Wednesday/Thursday/Friday/Saturday> gives the next possibilities
- Sunday
- Monday
- Tuesday
- Etc...
Checking for Errors
After making an API call, always check the error JSON field first. If this is empty, it is safe to proceed. See the Troubleshooting section below for more information.
A listing of the datasets that are returned for each of the methods can be found in our sandbox area under the API test area.
APIs Available
API V3.2 can be used for both Web and Agent bookings. The RCM API and Agent API allow you to make unallocated bookings for a specified vehicle category, for a specified pickup and drop off date. An unallocated booking is a booking that has been made for a particular category (e.g. small car), but has not been allocated to a particular vehicle. Allocation of a booking to a specific vehicle is done by the rental car company.
TroubleShooting
The first stage of your testing should be to make sure that your key is valid and working. You can do this at sandbox.rentalcarmanager.com/developer/livetest_3_2. Select your key from the list and click the Test
button. You should receive a message saying “The key works!”. If you don’t, or if there is an error returned,
then please contact RCM Support (support@rentalcarmanager.com)
as it is possible that there is an issue with your key.
If you receive an error "Access denied due to invalid subscription key" with 401 response code, check the following:
1. Check you are using the correct URL:
If you are calling the Agent API it should be
https://apis.rentalcarmanager.com/agent/booking/v3.2/?apikey=<apikey>2. Check you are using a "POST" request
3. Check that you are using the full API Key as provided, not url-encoded.
Any errors generated from your API calls will be returned in the “errors” field in your response.
If you are having problems with the API please ensure that you include debugging information in your email.
For server side debugging, include the full request, and details of the response that you are getting from our API server.
For client side debugging, you can use the developer tools in your browser. Here is an example of the network tool in Firefox showing an API call to step 2:
For more information about browser developer tools go to:
- Internet Explorer: How to use F12 Developer Tools to Debug your Webpages
- Firefox: Firefox Developer Tools
- Chrome: Chrome DevTools
Google Analytics Ecommerce Tracking
For those developers that want to add Google Analytics Ecommerce tracking to the web booking module, here is some sample code to show how you can get the booking information and send it on to Google.
Google Tag Manager
- Add code in the $(document).ready function to get the booking details and then call the function that creates the GTM datalayer:
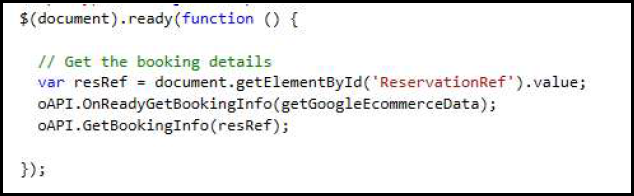
- Create the dataLayer object and send it to GTM:
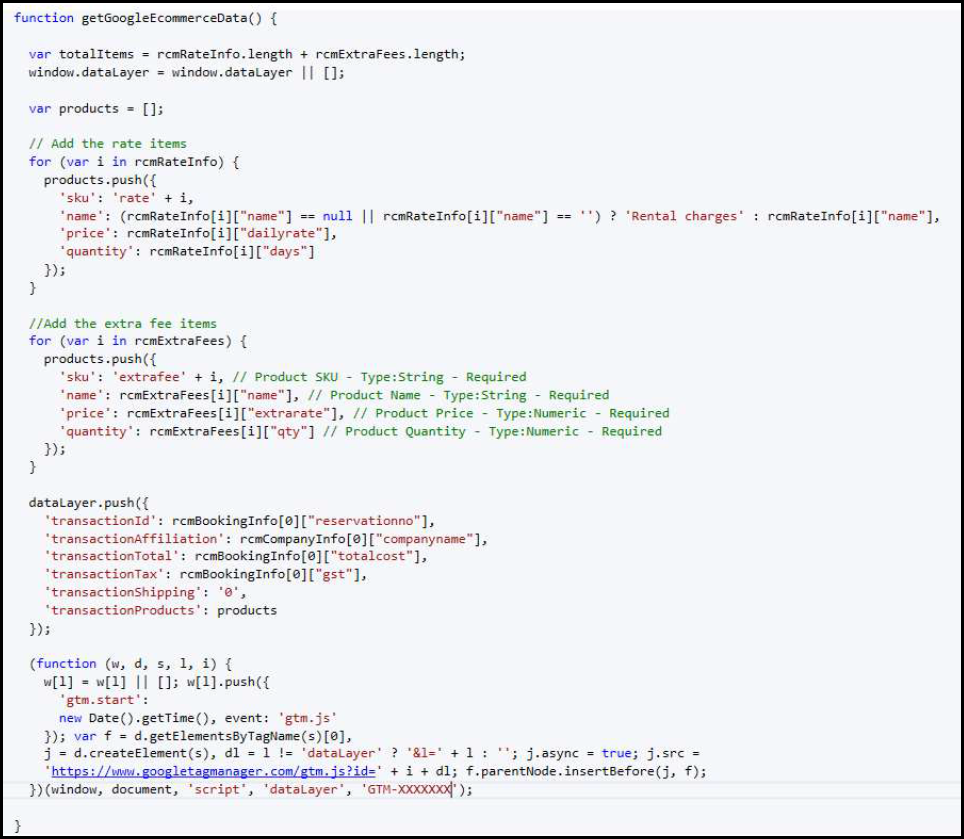
Google Analytics
- Add code in the $(document).ready function to get the booking details and then call the function that creates the GA transaction:
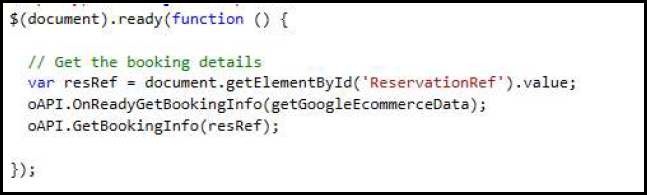
- Add the transaction and items to the GA object and send it to Google:
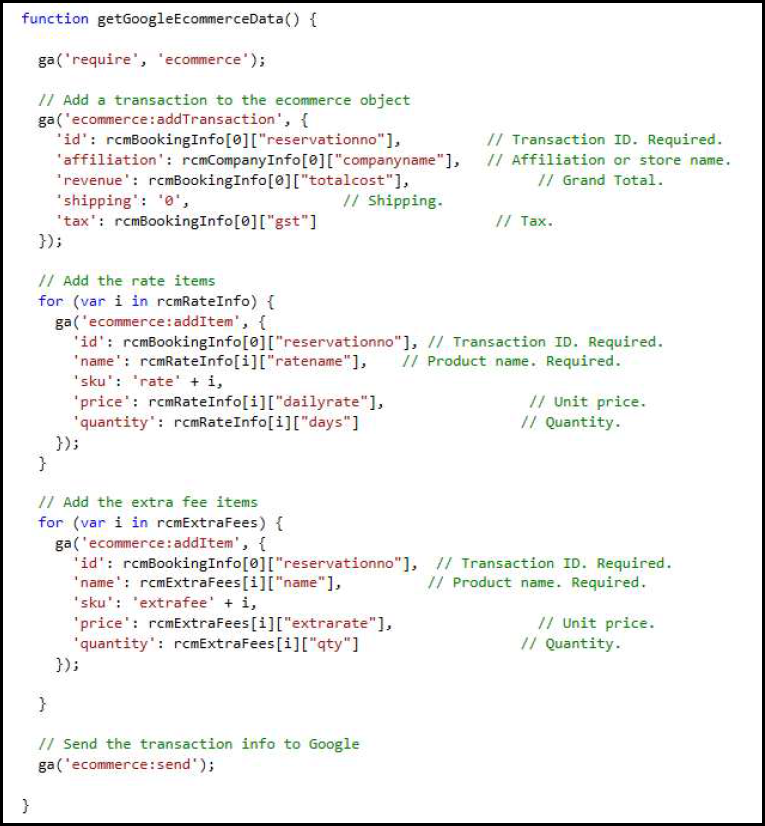